Data Structures & Sub-Programs (Edexcel GCSE Computer Science)
Revision Note
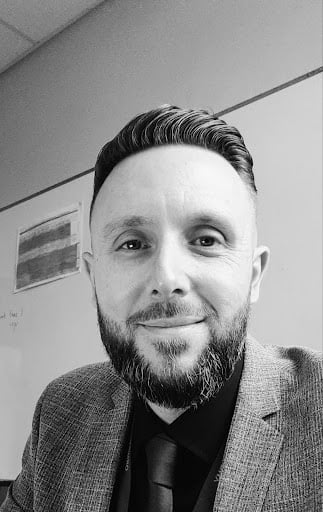
Author
Robert HamptonExpertise
Computer Science Content Creator
Data Structures
What is a data structure?
A data structure provides a structured way to store data, which makes it easy to access and manipulate
Examples of data structures are:
Arrays
Records
Arrays
An array is a sequence of data, of the same data type stored under the same identifier
Elements stored in an array can be identified by an index (position in the array)
Indexes start with the value 0
An array can be both 1-dimensional and 2-dimensional
Records
A record is a sequence of data, usually of mixed data types with a fixed length
Elements stored in an array can be identified by an index (position in the array)
Indexes start with the value 0
Structure | Example |
---|---|
Arrays |
|
Records |
|
scores[0]
holds the value 1scores[2]
holds the value 2students[3]
holds the value "Birmingham"
Exam Tip
Python has it's own data structure called a 'list' which can look like both an array and record BUT they are not the same thing!
In the exam, if a list holds data of all the same type then it can be thought of as an array, if data is different types it can be thought of as a record
Python example |
---|
# Create an array of numbers
# Access elements by index
# Print the entire array
# Modify an element
# Print the modified array
# Loop through the array
|
1-dimensional & 2-dimensional arrays
Python example |
---|
# 1-dimensional array (list of numbers)
# Access elements by index
# Loop through the array
# 2-dimensional array
# Access elements by row and column index
# Print the entire 2D array
# Loop through the 2D array (accessing elements)
|
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?