Evaluating Programs (Edexcel GCSE Computer Science)
Revision Note
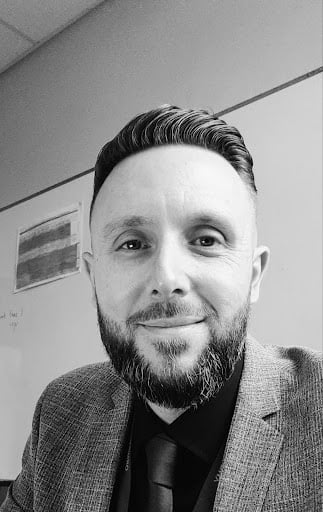
Author
Robert HamptonExpertise
Computer Science Content Creator
Fitness for Purpose & Efficiency
In the exam students should be able to demonstrate logical reasoning and use test data to evaluate a programs fitness for purpose & efficiency, this includes:
Number of compares
Number of passes through a loop
Use of memory
Number of compares
Python code |
---|
""" Finds the largest number in a list Inputs: numbers: A list of numbers Returns: The largest number in the list """ # Initialize largest number to the first element of the list # Iterate through the list, comparing each element with the current largest
# ------------------------------------------------------------------------ # Main program # ------------------------------------------------------------------------
|
This program uses a linear search algorithm to find the largest number
It iterates through each item in the list, comparing each element with the current largest value
For every comparison after the largest number is found, the comparison is unnecessary, making this method inefficient
As the number of elements grow, this method becomes increasingly more inefficient
This could be solved in Python by using a built in function such as
max()
which is optimised for finding the largest number
Number of passes through a loop
Python code |
---|
""" This function finds the target element in a list using a nested loop Inputs: data: A list of elements. target: The element to search for. Returns: The index of the target element in the list, or -1 if not found. """
# ------------------------------------------------------------------------ # Main program # ------------------------------------------------------------------------
|
This program uses nested loops to search for a value in a list of values
The outer loop iterates through each element in the list, the inner loop also iterates through each element
The inner loop checks if the current element is equal to the target
This approach is inefficient because the inner loop iterates through the entire list for every element in the outer loop
This program could be optimised by using the
in
operator
Use of memory
Python code (inefficient) |
---|
""" This function calculates an approximation of pi using a list Inputs: iterations: The number of iterations for the pi approximation. Returns: An approximation of pi. """
# Initialise a list to store all calculations
# Perform calculation and append to the list
# Calculate the average of elements
# ------------------------------------------------------------------------ # Main program # ------------------------------------------------------------------------
|
Python code (efficient) |
---|
""" This function calculates an approximation of pi Inputs: iterations: The number of iterations for the pi approximation. Returns: An approximation of pi. """
# Initialise variables to accumulate sum
# Perform calculation and update variables directly
# Calculate pi using the accumulated values
# ------------------------------------------------------------------------ # Main program # ------------------------------------------------------------------------
|
In the first program, pi is approximated by carrying out multiple calculations and storing the results in a list
This approach can lead to large lists being created, consuming large amounts of memory
In the second program, the same result is achieved by using two variables:
numerator
anddenominator
to accumulate the necessary values for the pi calculationThis approach avoids a large list and directly updates variables, leading to significantly lower memory usage
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?