Assembly Language & Little Man Computer (OCR A Level Computer Science)
Revision Note
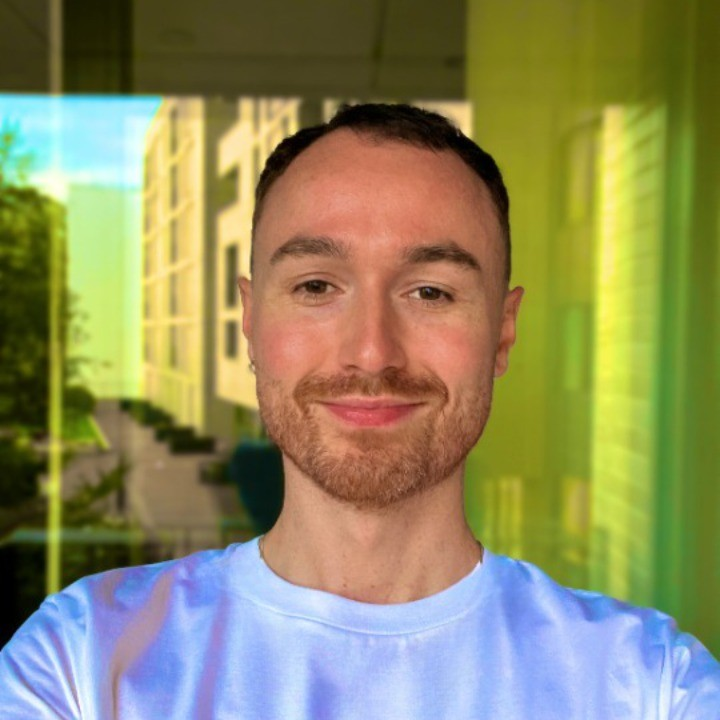
Author
Callum DaviesExpertise
Computer Science
Assembly Language & Little Man Computer
What is the Purpose of Assembly Language?
Assembly language sits between high-level languages (like Python, Java) and machine code (binary code executed by the computer hardware)
Allows developers to write more efficient, albeit more complex, code when compared to high-level languages
Direct access and manipulation of hardware components, e.g., registers, memory addresses
Each type of computer CPU has its specific assembly language
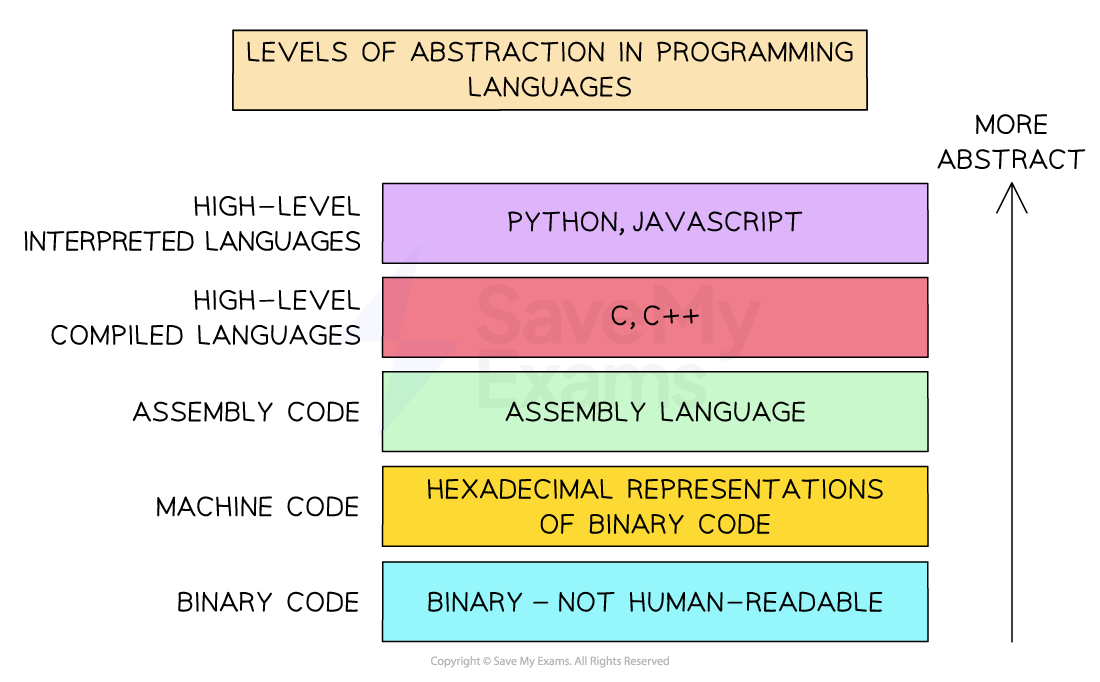
Levels of Abstraction of Programming Languages
Little Man Computer
The Little Man Computer (LMC) is a hypothetical computer model used for understanding the fundamental operations and mechanics of a computer
The LMC is a simplified version of a computer
It has key elements like memory, a calculator, an accumulator, and an instruction set
Little Man Computer Instruction set
The following mnemonics represent different actions that can take place in an LMC program
Mnemonic | Instruction | Alternative Mnemonic |
---|---|---|
ADD | Add |
|
SUB | Subtract |
|
STA | Store | STO |
LDA | Load | LOAD |
BRA | Branch always | BR |
BRZ | Branch if zero | BZ |
BRP | Branch if positive OR zero | BP |
INP | Input | IN, INPUT |
OUT | Output |
|
HLT | End program | COB, END |
DAT | Data location |
|
Example 1: Add two numbers
INP; // Input the first number
STA 90; // Store the first number in memory location 90
INP; // Input the second number
ADD 90; // Add the number in memory location 90 to the accumulator
OUT; // Output the result
HLT; // End the program
DAT; // Memory location 90 for storing data
Example 2: Find the smallest of three numbers
This program inputs three numbers and determines the smallest of the three, outputting the result.
INP // Input the first number
STA 91 // Store the first number in memory location 91
INP // Input the second number
STA 92 // Store the second number in memory location 92
INP // Input the third number
STA 93 // Store the third number in memory location 93
LDA 91 // Load the first number
SUB 92 // Subtract the second number
BRP CHECK_THIRD_FROM_FIRST // If result is positive, then first number > second number
LDA 92 // Load the second number
SUB 93 // Subtract the third number
BRP OUTPUT_SECOND // If result is positive, then second number > third number
LDA 93
OUT // Output the third number
HLT
CHECK_THIRD_FROM_FIRST:
LDA 91
SUB 93
BRP OUTPUT_FIRST
LDA 93
OUT
HLT
OUTPUT_FIRST:
LDA 91
OUT
HLT
OUTPUT_SECOND:
LDA 92
OUT
HLT
DAT // Memory locations for data storage
DAT
DAT
Worked Example
A digital thermostat has a CPU that uses the Little Man Computer Instruction Set.
The thermostat allows users to set a desired room temperature. The acceptable range for room temperature settings is between 15 and 25 degrees Celsius, inclusive. If the user sets a temperature within the range, the code outputs a 1 indicating a valid setting. If the temperature setting is outside of the acceptable range, the code outputs a 0 indicating an invalid setting.
The code is as follows:
INP
STA tempSetting
LDA minTemp
SUB tempSetting
BRP checkMax
LDA invalid
BRA end
checkMax
LDA maxTemp
SUB tempSetting
BRZ valid
BRP invalid
valid LDA valid
end OUT
HLT
valid DAT 1
invalid DAT 0
minTemp DAT 15
maxTemp DAT 25
tempSetting DAT
a) What is the purpose of the label checkMax in the code? Describe its role in determining the validity of the temperature setting.
[2]
b) If a user inputs a temperature setting of 14, what will be the output? Justify your answer.
[2]
c) If the acceptable range of temperature settings was expanded to include temperatures up to 30 degrees Celsius, what modification would be needed in the code?
[2]
Answer:
Example answer that gets full marks:
a) The label checkMax begins a code segment that checks if the user's desired temperature is within the maximum allowable temperature. It subtracts the user's input from the maximum temperature (maxTemp). If the result is zero (meaning they are equal) or positive (meaning the user's input is less than maxTemp), the user's desired temperature is within the allowable range.
b) If a user inputs a temperature setting of 14, the output will be 0 (indicating an invalid setting). This is because when 14 is subtracted from the minimum allowed temperature (minTemp), the result is positive, which then causes the code to skip checking the maximum value and directly output the invalid value, which is 0.
c) To increase the maximum allowable temperature setting to 30 degrees Celsius, modify the maxTemp DAT value. The new line should read:
maxTemp DAT 30
Acceptable answers you could have given instead:
a) Any response mentioning that checkMax it is for checking if the user's input is below or equal to the maximum allowable temperature should be awarded marks.
b) Any answer stating that the output will be 0 because 14 is less than 15, or similar logic, should be awarded marks.
c) Any answer suggesting a change to the maxTemp DAT value to 30 should be awarded marks.
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?