Selection in JavaScript (OCR A Level Computer Science)
Revision Note
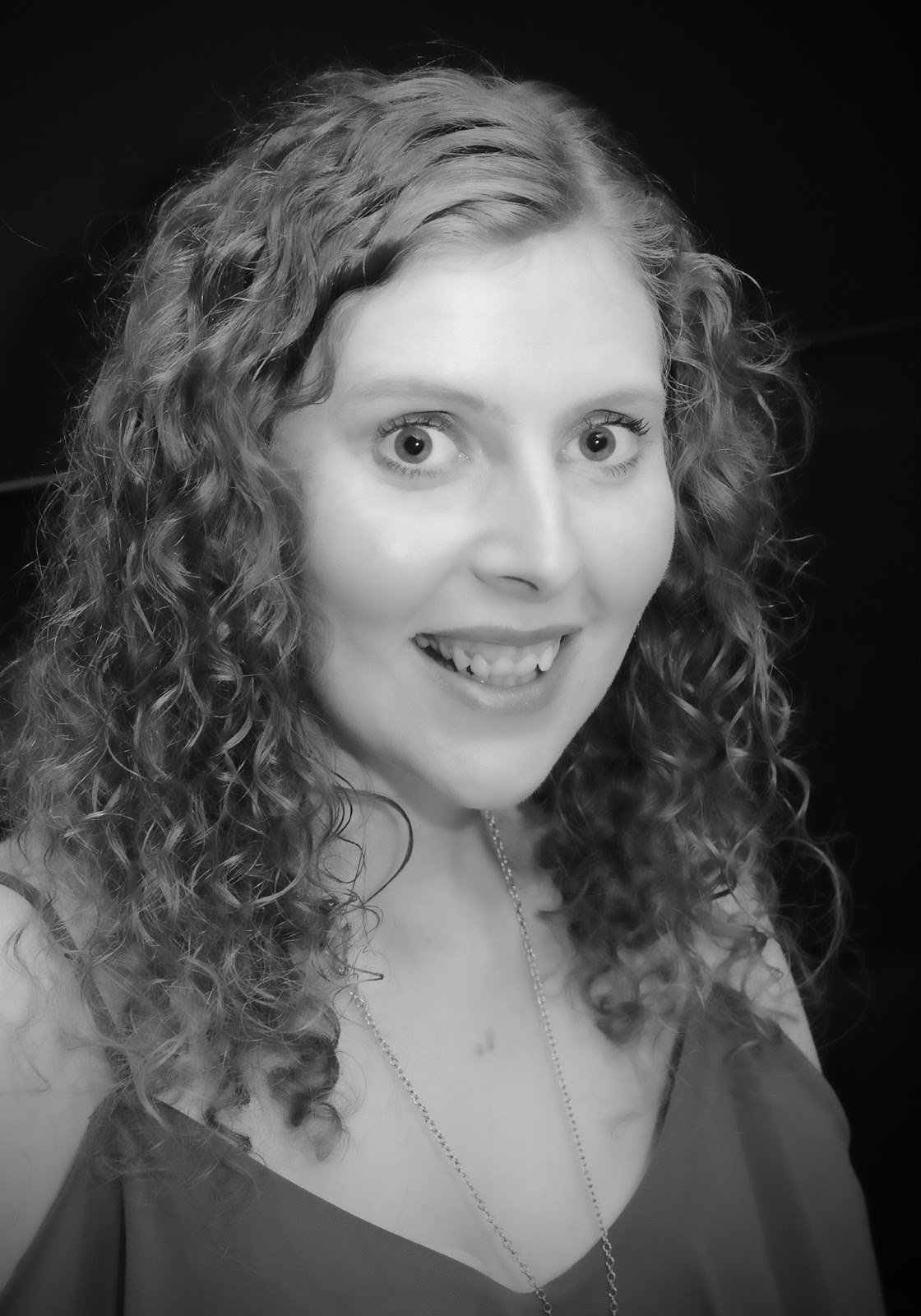
Author
Becci PetersExpertise
Computer Science
Selection in JavaScript
Programming code is made up of constructs which control the flow of a program
Constructs tell the computer the order in which to carry out the statements/lines of code
There are 3 programming constructs:
Sequence
Selection
Iteration
Selection is selecting a line/lines of code to run depending on whether a condition is true or false
There are two ways to write selection statements:
If
statementsSwitch case
statements
When writing a condition there will need to be a comparison operator. They are listed below:
Operator | Description | Example (where x=5) | Returns |
---|---|---|---|
== | equal to | x == 8 | false |
x == 5 | true | ||
x == "5" | true | ||
=== | equal value and equal type | x === 5 | true |
x === "5" | false | ||
!= | not equal | x != 8 | true |
!== | not equal value or not equal type | x !== 5 | false |
x !== "5" | true | ||
x !== 8 | true | ||
> | greater than | x > 8 | false |
< | less than | x < 8 | true |
>= | greater than or equal to | x >= 8 | false |
<= | less than or equal to | x <= 8 | true |
IF Statements in JavaScript
An
if the
statement will let you choose a line/lines of code to run if a condition is true or falseBelow are three examples of
if
statements:
if
if else
if else if else
Syntax of an if statement
The syntax of an if
the statement consists of the if
keyword, followed by a condition enclosed in brackets, and a code block that is executed if the condition evaluates to true
:
if (condition) {
// Code to be executed if the condition is true
}
Pseudocode example of an if statement
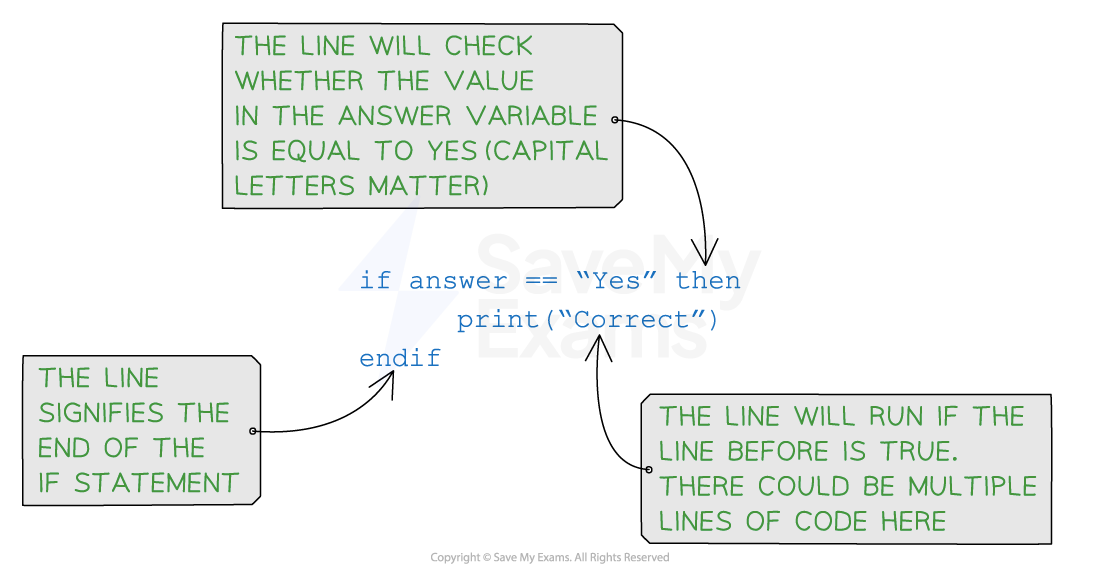
Example in JavaScript: checking if a number is positive
const number = 5;
if (number > 0) {
console.log('The number is positive.');
}
In this example, the
if
statement checks if the value of the variablenumber
is greater than0
. If the condition is true, the message'The number is positive.'
is output to the browser
Syntax of an if-else statement
The if the
statement can be extended with an else
clause to specify an alternative block of code that is executed when the condition evaluates to false
:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Pseudocode example of an if-else statement
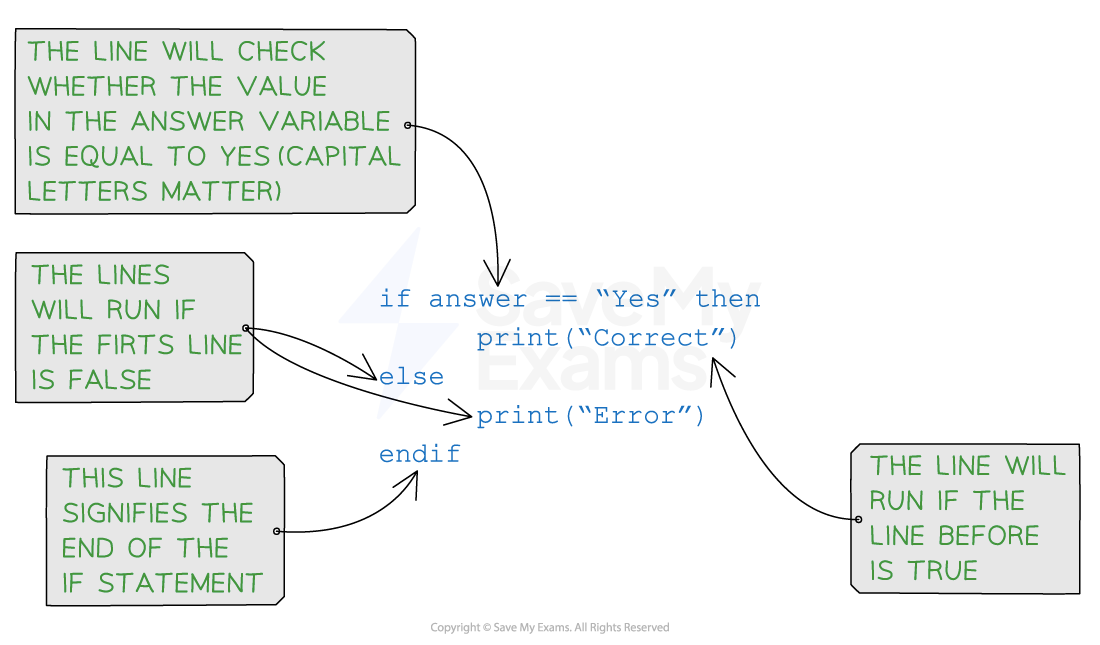
Example in JavaScript: Checking if a number is positive or negative
const number = -3;
if (number > 0) {
console.log('The number is positive.');
} else {
console.log('The number is not positive.');
}
In this example, if the value of
number
is greater than0
, the message'The number is positive.'
is output. Otherwise, the message'The number is not positive.'
is output
Syntax of an If Else-If Else statement
The else if
the clause specifies additional conditions to check if the initial if
condition is false. This allows the handling of multiple scenarios in a more complex decision-making process:
if (condition) {
// Code to be executed if the condition is true
} else if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Pseudocode example of an If Else-If Else statement
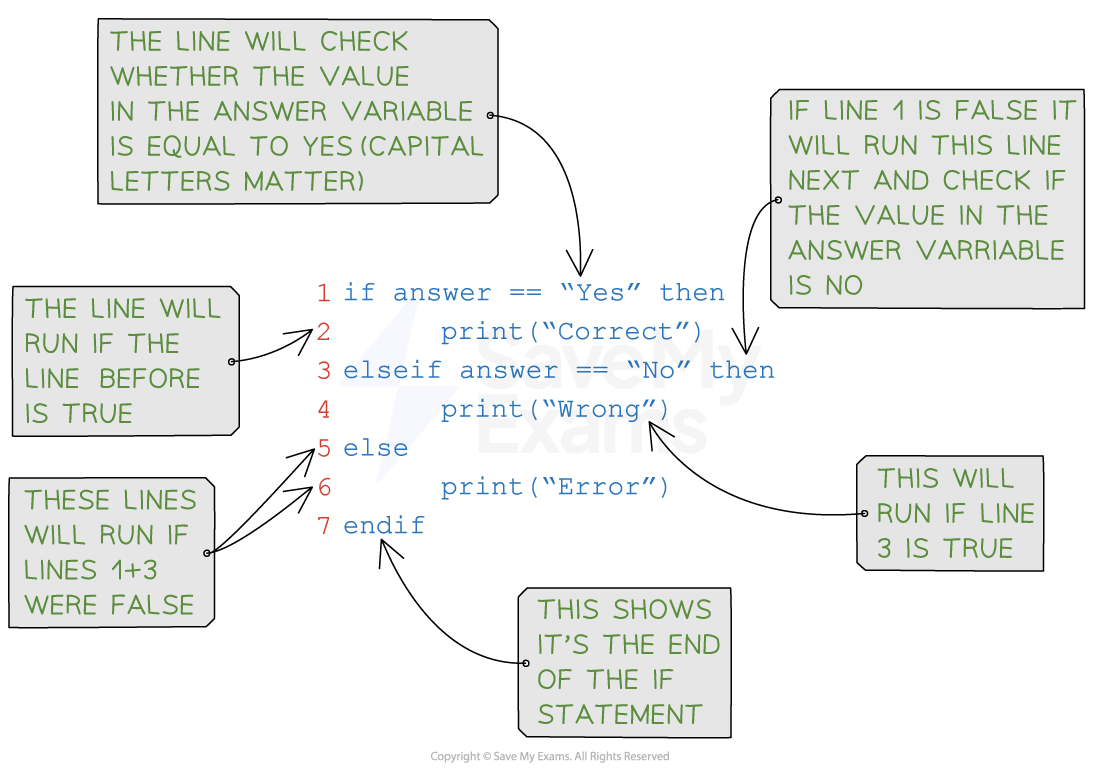
Example in JavaScript: Grading a score
const score = 85;
if (score >= 90) {
console.log('Excellent!');
} else if (score >= 80) {
console.log('Good.');
} else if (score >= 70) {
console.log('Fair.');
} else {
console.log('Needs improvement.');
}
In this example, the
if-else if-else
statement evaluates the value ofscore
to determine the corresponding message based on the score range
Exam Tip
You can use as many
else if
s as you want to within yourif
statement but it might be clearer to use aswitch case
statementYou can have an
if else-if
statement without an end as the catch-all condition at the end
How do I write my condition?
Think of it like writing a yes/no question
E.g.
Is the number bigger than 10?
Is the number between 50 and 100?
Is the answer Paris?
If the question can’t be answered with yes/no then it needs to be rewritten in this way
Is it possible to use more than one operator?
Yes! Using one operator is most common but sometimes 2 are needed. More than 2 could be used but it gets more complicated. This involves using boolean operators
Imagine a program where the user has to enter a number based on the role of a dice. The number needs to be between 1 and 6
The yes/no question could be is the number between 1 and 6 - but it would be structured slightly differently in the code
IF number >=1 AND number <=6 then
The first check is if the number is greater than or equal to 1
The second check is if the number is less than or equal to 6
The final check is if both sides are True
Exam Tip
Check whether to use an
AND
or anOR
in the condition - it’s easy to get these 2 mixed up. E.g.IF number<0 AND number>100
will check if the number is below 0 and greater than 100 (there are no numbers which fit into this range!)IF number<0 OR number>100
will check if the number is between 1 and 99
Worked Example
A website sells tickets for sporting events. The website uses HTML, CSS and JavaScript. The website charges a booking fee of £2.99 on each ticket sold. In addition, if the tickets are purchased from outside of the UK, £4.99 is added to the booking fee. The booking fee is calculated using a JavaScript function named bookingfee()
.
Complete the definition of the bookingfee()
function below.function bookingfee(numtickets, country) {
var nonUKprice = 4.99;
var perTicketPrice = .............................................;
var total = 0;
if (country!="UK") {
total = total + .............................................;
}
total = total + (............................................. * perTicketPrice);
return total;
}
3 marks
How to answer this question:
The first blank space is to set the value of the
perTicketPrice
. The question tells us this is £2.99The 2nd blank space is to add something to
total
if the country is not equal to UK. The question tells us that if the tickets are purchased from outside the UK, £4.99 is added to the booking fee. This is stored in thenonUKprice
variableThe 3rd blank space is something multiplied by
perTicketPrice
. The question tells us each ticket is £2.99 so we need to multiply theperTicketPrice
by the number of tickets (callednumtickets
)
Answer:
Example answer that gets full marks:
function bookingfee(numtickets, country) {
var nonUKprice = 4.99;
var perTicketPrice = 2.99;
var total = 0;
if (country!="UK") {
total = total + nonUKprice;
}
total = total + (numtickets * perTicketPrice);
return total;
}
Exam Tip
When you're given code in a question that you need to refer to or complete, you must spell existing identifier names exactly as they have in the question. E.g. if you write
numtikets
instead ofnumtickets
you won't get the markAlso, make sure you don't include £ in the calculation - this will be added as an output and will cause the calculation to not work as it's included a character that's not an integer
Switch Case in JavaScript
Switch Case
is a type of conditional statement that provides an alternative way to perform multiple comparisons based on the value of an expressionThese statements are particularly useful when you have a single expression that you want to compare against multiple possible values
Syntax of a Switch Case statement
The syntax of a
switch case
statement consists of theswitch
keyword followed by an expression enclosed in bracketsThis expression is evaluated, and its value is then compared against various
case
labels. If a match is found, the corresponding block of code is executedThe
default
keyword is optional and specifies a block of code to be executed if none of the case labels match the expression:
switch (expression) {
case value1:
// Code to be executed if the expression matches value1
break;
case value2:
// Code to be executed if the expression matches value2
break;
// more case statements
default:
// Code to be executed if no case matches the expression
}
Switch case example in JavaScript: Grades evaluation
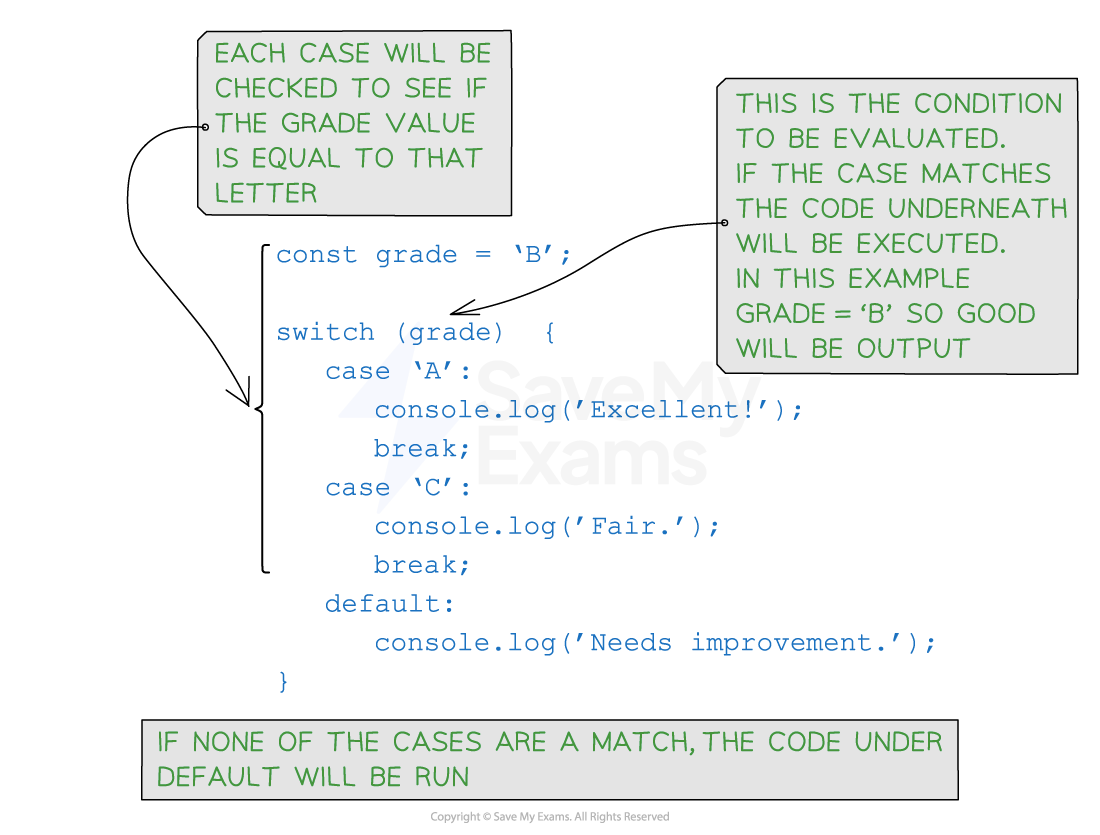
Fall-Through behaviour
By default, switch case
has a fall-through behaviour, meaning that if a case label matches, the code execution continues to the next case until a break
statement is encountered. This allows you to group multiple cases with the same code:
const day = 'Monday';
switch (day) {
case 'Monday':
case 'Tuesday':
case 'Wednesday':
case 'Thursday':
case 'Friday':
console.log('Weekday');
break;
case 'Saturday':
case 'Sunday':
console.log('Weekend');
break;
default:
console.log('Invalid day.');
}
In this example, if
day
is 'Tuesday', 'Weekday' is logged to the console because it falls through the cases of 'Monday' to 'Friday' until abreak
statement is encountered
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?