Nested Statements in JavaScript (OCR A Level Computer Science)
Revision Note
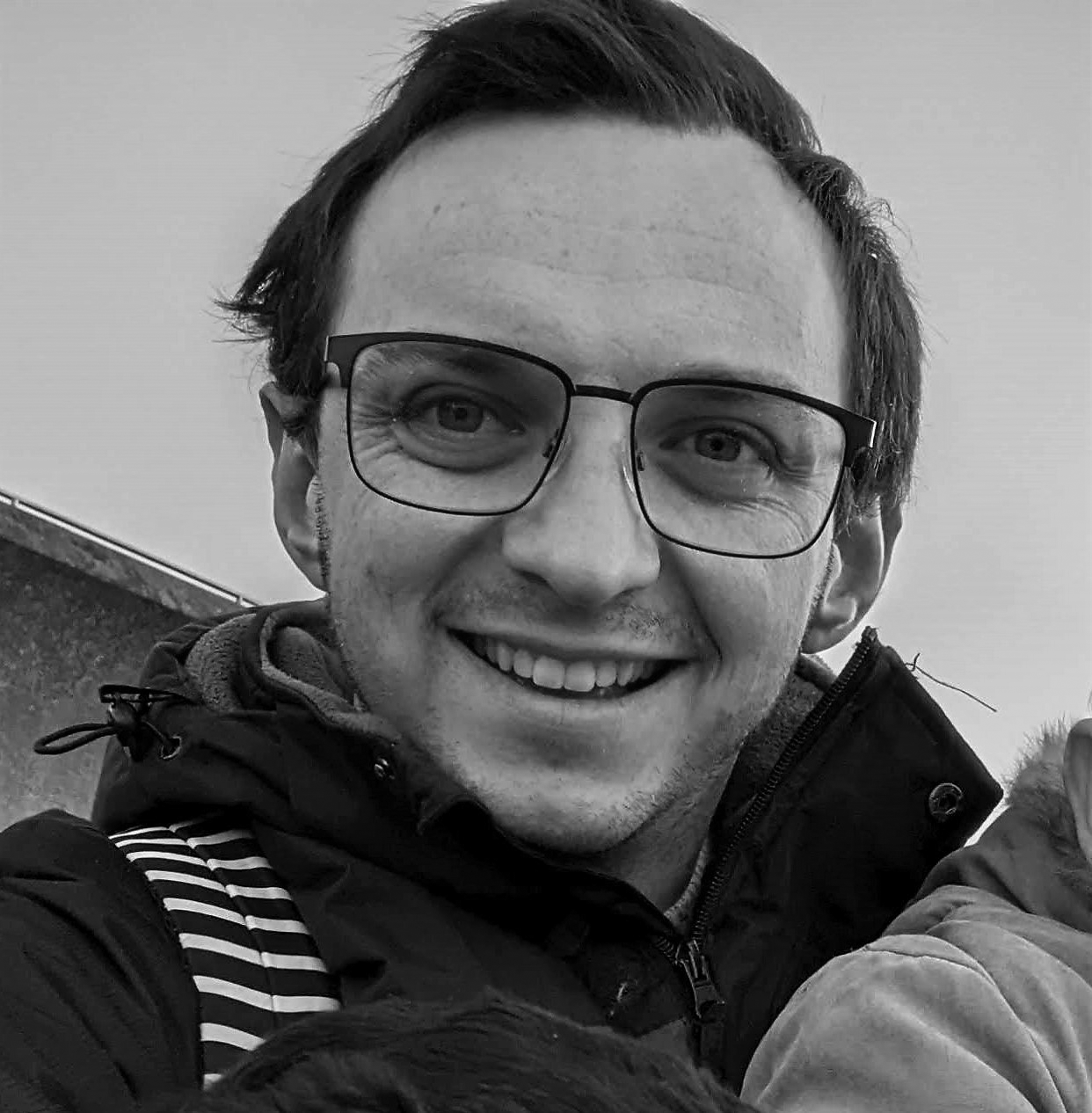
Author
Jamie WoodExpertise
Maths
Nested Statements in JavaScript
Nesting is putting one block of code within another. This is commonly done with if
statements and loops.
Nested
if
statements refer to the practice of including one or more conditional statements inside the block of another conditional statement. These nested structures allow you to create more complex decision-making logic by evaluating multiple conditions and handling various scenarios within your codeNested loops refer to the practice of including one or more loops inside the block of another loop. These nested structures allow you to iterate over multiple sets of data and create more complex patterns of repetition within your code
Nested If Statements in JavaScript
If
statements can be nested within each other to handle more intricate conditions and decision-making:
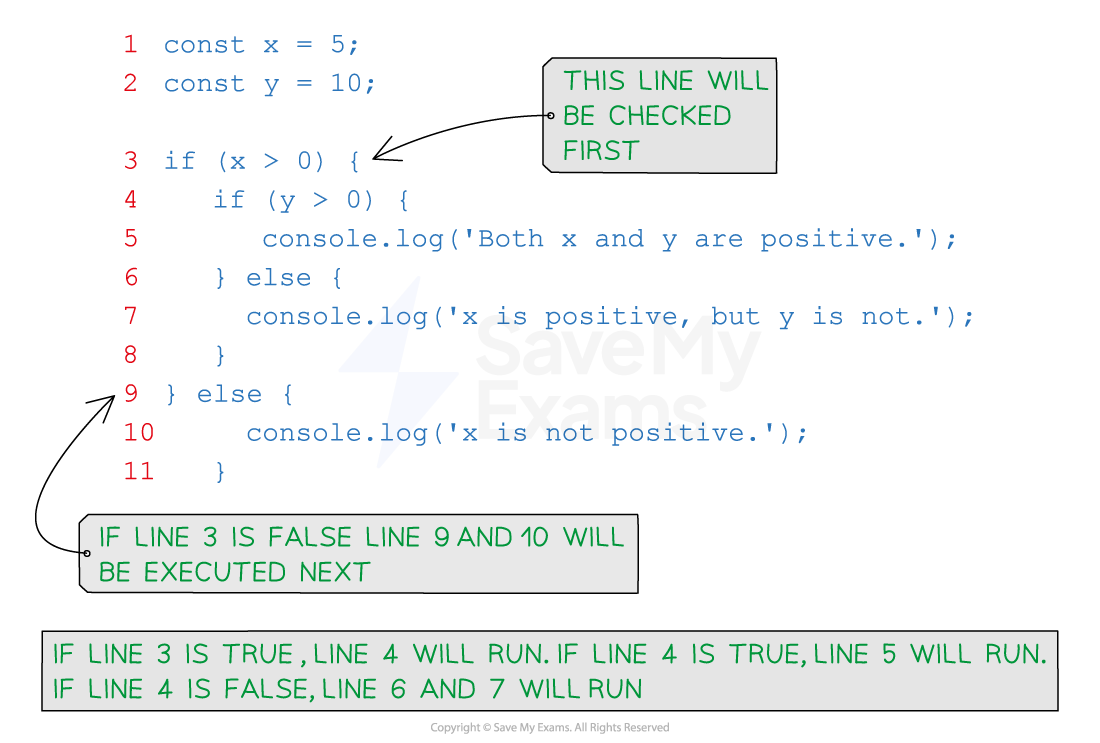
Nested if statement in JavaScript
Nested Loops in JavaScript
The syntax of nested loops involves placing one loop (for
loop, while
loop, etc.) inside the block of another loop:
for (let i = 0; i < outerArray.length; i++) {
// Code block for outer loop
for (let j = 0; j < innerArray.length; j++) {
// Code block for inner loop
// More nested loops if needed
}
// More code after inner loop
}
Example: Nested for loops
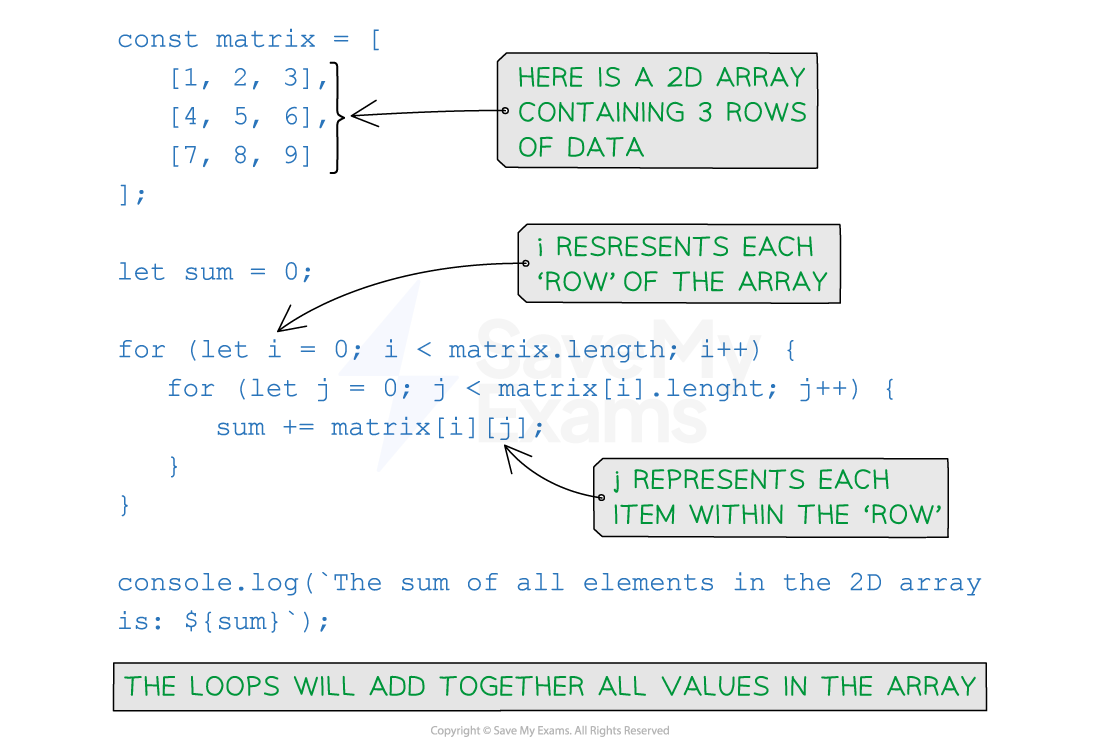
Nested for loop in JavaScript showing a 2D array with each item in the array being added together to find the sum of the whole array
After the first iteration
sum=1
as it's only added the 1st element which is 1After the 2nd iteration
sum=3
as it's added the 2nd element (2) to 1After the 3rd iteration
sum=6
as it's added the 3rd element (3) to the 1 and 2After the 4th iteration
sum=10
as it's added the 4th element (4) to the 1, 2 and 3The algorithm continues working its way through the rest of the 2nd row and then moves on to the 3rd
Example: Nested while loops
This code below does the same as the code above but utilises while
loops rather than for
loops:
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
let i = 0;
while (i < matrix.length) {
let j = 0;
while (j < matrix[i].length) {
console.log(matrix[i][j]);
j++;
}
i++;
}
Exam Tip
If you need to check or work out how many times an inner loop is run, multiply the number of outer loop iterations by the number of inner loop iterations. E.g.
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 5; j++) {
console.log(i * j);
}
}
The outer loop runs 3 times and the inner loop will run 5 times. To find out the total number of times the inner loop will run, multiply 3 by 5 = 15
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?