Records, Lists & Tuples (OCR A Level Computer Science)
Revision Note
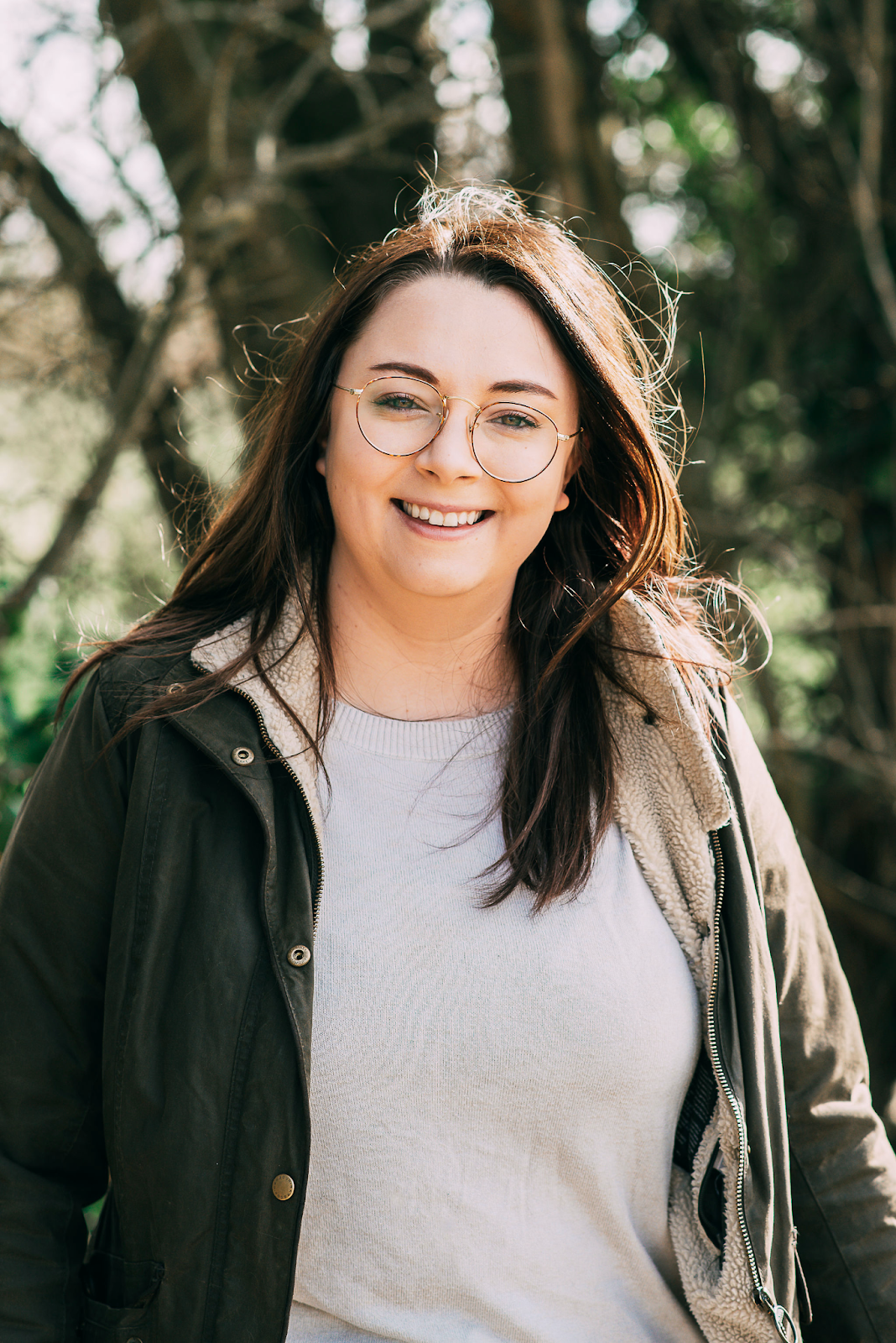
Author
Jennifer PageExpertise
Computer Science
Records
What is a Record?
A record is a row in a file and is made up of fields. They are used in databases as shown in the example below
ID | firstName | surname |
---|---|---|
001 | Tony | Smith |
002 | Peter | Pavard |
003 | Robert | Brown |
The above example contains 3 records, where each record has 3 fields. A record can be declared as follows
Example in Pseudocode
personDataType = record
integer ID
string firstName
string surname
endRecord
Example in Python
class Person:
def __init__(self, ID, firstName, surname):
self.ID = ID
self.firstName = firstName
self.surname = surname
# Creating an instance of the Person record
person_record = Person(1, "Tony", "Smith")
# Accessing the fields of the Person record
print("ID:", person_record.ID)
print("First Name:", person_record.firstName)
print("Surname:", person_record.surname)
In the code above, a class is defined called “Person” with an ‘__init__’ method that initialises the fields of the record
Create an instance of the “Person” record called ‘person_record’ with an ID of 1, first name “Tony”, and surname “Smith”
Finally access and print the values of the fields using dot notation (‘object.field’)
Example in Java
public class Person {
private int ID;
private String firstName;
private String surname;
public Person(int ID, String firstName, String surname) {
this.ID = ID;
this.firstName = firstName;
this.surname = surname;
}
public int getID() {
return ID;
}
public String getFirstName() {
return firstName;
}
public String getSurname() {
return surname;
}
public void setID(int ID) {
this.ID = ID;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setSurname(String surname) {
this.surname = surname;
}
}
Define a class called ‘Person’ with private fields for ID, firstName, and surname
Then a constructor that allows you to set these fields when creating an instance of the record
Additionally, provide getter and setter methods for each field to access and modify their values
To use the record in Java, create an instance of ‘Person’ class and set or retrieve the field values using the provided methods
For example:
public class Main {
public static void main(String[] args) {
Person personRecord = new Person(1, "Tony", "Smith");
// Accessing the fields of the Person record
int ID = personRecord.getID();
String firstName = personRecord.getFirstName();
String surname = personRecord.getSurname();
System.out.println("ID: " + ID);
System.out.println("First Name: " + firstName);
System.out.println("Surname: " + surname);
}
}
This creates an instance of the ‘Person’ record called ‘personRecord’ with an ID of 1, first name “Tony”, and surname “Smith”
Then uses the getter methods to retrieve the field values and print them to the console
Lists
What is a List?
A list is a data structure that consists of a number of items where the items can occur more than once
Lists are similar to 1D arrays and elements can be accessed in the same way. The difference is that list values are stored non-contiguously
This means they do not have to be stored next to each other in memory, which is different to how data is stored for arrays
Lists can also contain elements of more than one data type, unlike arrays
Example in Pseudocode
list pets = ["dog", "cat", "rabbit", "fish"]
Example in Python
pets = ["dog", "cat", "rabbit", "fish"]
Example in Java
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> pets = new ArrayList<>();
// Adding elements to the pets list
pets.add("dog");
pets.add("cat");
pets.add("rabbit");
pets.add("fish");
// Printing the elements in the pets list
for (String pet : pets) {
System.out.println(pet);
}
}
}
Worked Example
A programmer has written the following code designed to take in ten names then print them in a numbered list.
name1 = input("Enter a name: ")
name2 = input("Enter a name: ")
name3 = input("Enter a name: ")
name4 = input("Enter a name: ")
name5 = input("Enter a name: ")
name6 = input("Enter a name: ")
name7 = input("Enter a name: ")
name8 = input("Enter a name: ")
name9 = input("Enter a name: ")
name10 = input("Enter a name: ")
print("1. " + name1)
print("2. " + name2)
print("3. " + name3)
print("4. " + name4)
print("5. " + name5)
print("6. " + name6)
print("7. " + name7)
print("8. " + name8)
print("9. " + name9)
print("10. " + name10)
It has been suggested that this code could be made more efficient and easier to maintain using an array or a list.
Write a more efficient version of the programmer’s code using an array or a list.
5 marks
How to answer this question:
Start by having a variable names which is initialised as an empty list []
names = []
Then have a for loop run from i = 0 to i = 9 (inclusive), indicating that it will iterate 10 times
for i = 0 to 9
Inside the loop, the input() function is used to prompt the user to enter a name. The entered name is then appended to the names list using the append() method
names.append(input(“Enter a name: “))
After the first for loop finishes executing, the list names will contain 10 names entered by the user
Then a second for loop also runs from i = 0 to i = 9 (inclusive)
for i = 0 to 9
Inside this loop, the print() function is used to display the index of each name (incremented by 1) along with the name itself. The expression i + 1 represents the current index, and (i + 1) + ". " is concatenated with names[i] to form the output string
print((i+1)+”. “+names[i])
The loop repeats this process for each name in the names list, printing the index number and corresponding name on separate lines
Answer:
Example answer that gets full marks:
names = []
for i = 0 to 9
names.append(input(“Enter a name: “))
next i
for i = 0 to 9
print((i+1)+”. “+names[i])
next i
Declaration of list/array [1]
For loop with runs ten times [1]
Inputting name to correct location each interaction [1]
For/while loop which outputs each name [1]
Names are formatted with numbers 1-10 and a dot preceding each one [1]
Manipulating Lists
There are a range of operations that can be performed involving lists in the table below:
List Operation | Description | Pseudocode |
---|---|---|
isEmpty() | Checks if the list is empty |
|
append(value) | Adds a new value to the end of the list |
|
remove(value) | Removes the value the first time it appears in the list |
|
length() | Returns the length of the list |
|
index(value) | Returns the position of the item |
|
insert(position, value) | Inserts a value at a given position |
|
pop() | Returns and removes the last value |
|
Tuples
What is an Tuple?
A tuple is an ordered set of values of any type
It is immutable, this means that it can’t be changed
Elements, therefore, can’t be added or removed once it has been created
Tuples are initialised using regular brackets instead of square brackets
Elements in a tuple are accessed in a similar way to elements in an array, with the exception that values can’t be changed
Example in Pseudocode
tuple1 = (1, 2, 3)
Example in Python
person = ("John", 25, "USA")
print(person)
When should Arrays, Records, Lists & Tuples be used?
Data Structure | Appropriate Usage | Possible use cases |
---|---|---|
Array | A fixed-size collection of elements and need random access to elements inside by index. They are efficient for accessing elements by index but less efficient for dynamic resizing of insertion/removal of elements in the middle. | List of student grades A table of numbers for a mathematical function A grid of pixels for an image |
Records | A group of related data which is a single entity. They are useful for organising and manipulating data with different attributes of fields. | A shopping list A to-do list A list of contacts in an address book |
Lists | A dynamic collection of elements that may change in size. They provide flexibility for adding, removing and modifying elements. | A customer record in a database A student record in a school system A product record in an online store |
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?