Pipelining as a Computational Method (OCR A Level Computer Science)
Revision Note
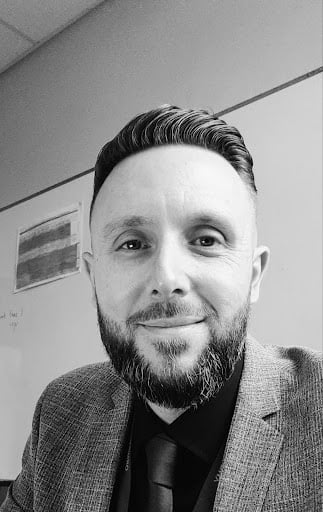
Author
Robert HamptonExpertise
Computer Science Content Creator
Pipelining as a Computational Method
Before revising pipelining as a computational method, it is important to revise the concept of pipelining within the CPU first.
What is Pipelining?
Pipelining is the process of carrying out multiple instructions concurrently
It improves the overall efficiency and performance of a task or instruction
In order to utilise pipelining it can be broken down into the following stages:
Break down the task - analyse the process carefully to identify the individual tasks that make up the overall job
Arrange in sequential order - organise the tasks into a logical sequence, where the output of one task becomes the input for the next
Allow multiple tasks to operate at the same time - instead of waiting for one task to fully complete before starting the next one, allow multiple tasks to operate concurrently
Pipelining in Programming
In languages such as Python and Java pipelining is chaining multiple instructions together
Pipelining is a similar concept to an assembly line which is used to make the overall process more efficient
Here is an example program written in Python that generates squared values of numbers from a list and then filters them to find only the even values
01 list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
02 squared = [x**2 for x in list]
03 filtered = [x for x in squared if x % 2 == 0]
04 print(filtered)
Line 01 creates a list of ten numbers
Line 02 uses iteration to square each value in the list one by one and generate a new list called ‘squared’
Line 3 filters the new list, searching for each even number and adding them to a separate list called "filtered"
On line 04 we print the filtered list, the final output would be:
[4, 16, 36, 64, 100]
In the first example commands are separated by being on their own individual numbered lines, in other languages such as Unix the ‘|’ symbol is used to separate commands
Each command in the ‘pipeline’ is separated and data flows left to right
An example of the ‘|’ symbol used in Unix:
grep “hello” docs | grep “world”
Here two
grep
commands (search) are chained together to perform a specific taskThe first
grep
commandgrep “hello” docs
searches for lines that contain the word “hello” in a list of files contained in a file named ‘docs’The pipe symbol ‘|’ connects the output of the first command to the input of the second command meaning only lines containing the word “hello” are passed to the second command
The second
grep
commandgrep “world”
searches for lines in the filtered results from command 1 that contain the word “world”
Worked Example
When a car is manufactured, the following activities take place:
Designs drawn
Engine developed
Chassis developed
Electronics planned
Welding to create the body of the car
Painting to protect the cars exterior
Engine assembled
Interior assembly
Wheels attached
Final inspection
Define the term ‘pipelining’ [2]
Carrying out instructions concurrently [1]
The result from one process/procedure feeds into the next [1]
Explain how the principles of pipelining can be applied to ensure a car is manufactured as quickly as possible [4]
Identify tasks that can be carried out at the same time [1] for example, the engine and chassis can be developed separately but at the same time once the designs have been drawn [1]
Identify tasks that must be completed in order [1] for example, painting once the body has been created/final inspection once all other tasks have been completed [1]
Describe one example of where pipelining is user in a computer system [2]
Pipes to pass data between programs [1] for example, the | symbol used in Unix [1]
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?