Modes of Addressing (OCR A Level Computer Science)
Revision Note
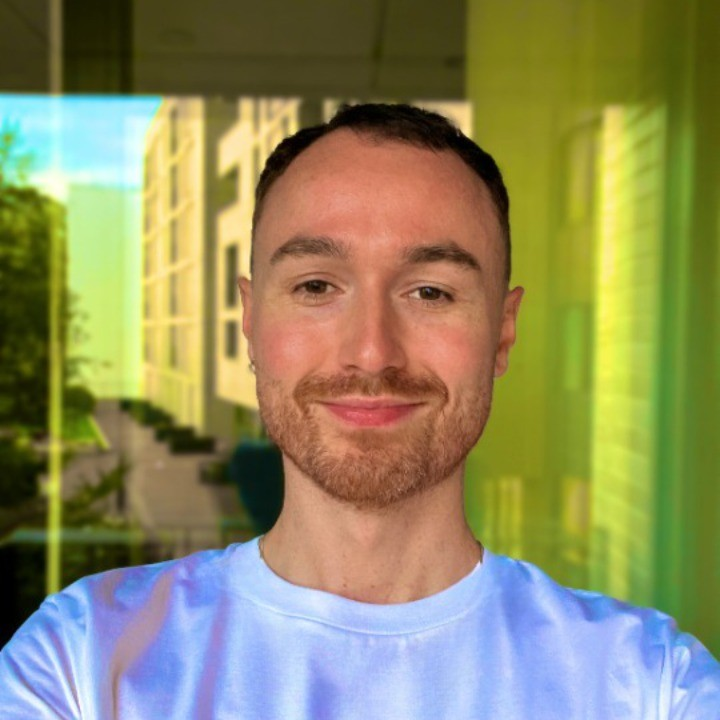
Author
Callum DaviesExpertise
Computer Science
Modes of Addressing
Immediate Addressing
Operand is part of the instruction itself
MOV AX, 1234h // Moves the immediate hex value 1234h to the AX register
Direct Addressing
The memory address of the operand is directly specified
MOV AX, [1234h] // Take the value stored in memory location 1234h and move to the AX register
Indirect Addressing
A register contains the memory address of the operand
If
BX
contains the value2000h:
MOV AX, [BX] // Moves the value from memory location 2000h to the AX register
This does not mean "Move the value 2000h into AX"
Instead, it means, "Look in the memory address 2000h (the value currently stored in the BX register) and move whatever value you find into the AX register."
When brackets
[ ]
are around a register in assembly language (like[BX]
), it's an instruction to treat the value inside that register as a memory address and to use the data at that memory address for the operationIndexed Addressing
Combines a base address with an index to compute the effective address
If
BX
contains0050h
andSI
has a base address1000h
:
MOV AX, [BX + SI] // Move the value at memory location 1050h to AX
Fetches data from the effective address (because
1000h + 0050h
is1050h
) and moves it into the AX register
Worked Example
Consider a basic computer system with the following assembly language instructions and a memory layout starting at address 1000
.
1000: MOV AX, 8
1002: ADD AX, [BX]
1004: MOV [0008], AX
1006: MOV CX, [BX+DI]
1008: HLT
Assume the registers have the following values before execution:
AX = 0000
BX = 0003
DI = 0002
CX = 0010
Memory contains:
0000: 0
0001: 0
0002: 0
0003: 5
0004: 0
0005: 7
0006: 7
0007: 9
0008: 0
a) For the instruction at 1002
, identify the addressing mode used and explain what it does in the context of this instruction.
[2]
b) After the instruction at 1004
has executed, what will the value at memory address 0008
be? Justify your answer.
[2]
c) What value will be moved into the CX
register after the instruction at 1006
executes? Explain the addressing mode used.
[2]
Answer:
Answer that gets full marks:
a) The instruction at 1002
uses Indirect Addressing. The instruction ADD AX, [BX]
adds the value at the memory address contained in the BX
register to the AX
register. In this case, since BX
is 3
, it will add the value at memory address 3
to AX
.
b) The value at memory address 0008
will be 13
. Before the instruction, AX
contains the value 8
. After adding 5
(from memory address 3
due to the instruction at 1002
), AX
will have the value 13
. The instruction at 1004
then moves this value to memory address 0008
.
c) The value moved into the CX
register will be 7
. The instruction at 1006
uses Indexed Addressing. It accesses memory by combining the address in BX
with the offset in DI
. Given BX
is 3
and DI
is 2
, the effective address is 3 + 2 = 5
, so it fetches the value 7
from address 0005
into CX
.
Acceptable responses:
a) Any answer identifying Indirect Addressing and explaining its use in the context of fetching a value from memory for the instruction should be awarded marks.
b) Any answer stating that the value at address 0008
will be 13
due to adding 8
and 5
should be awarded marks.
c) Any response indicating the use of Indexed Addressing and explaining the value fetch from address 5
should be awarded marks.
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?