Functions & Procedures in JavaScript (OCR A Level Computer Science)
Revision Note
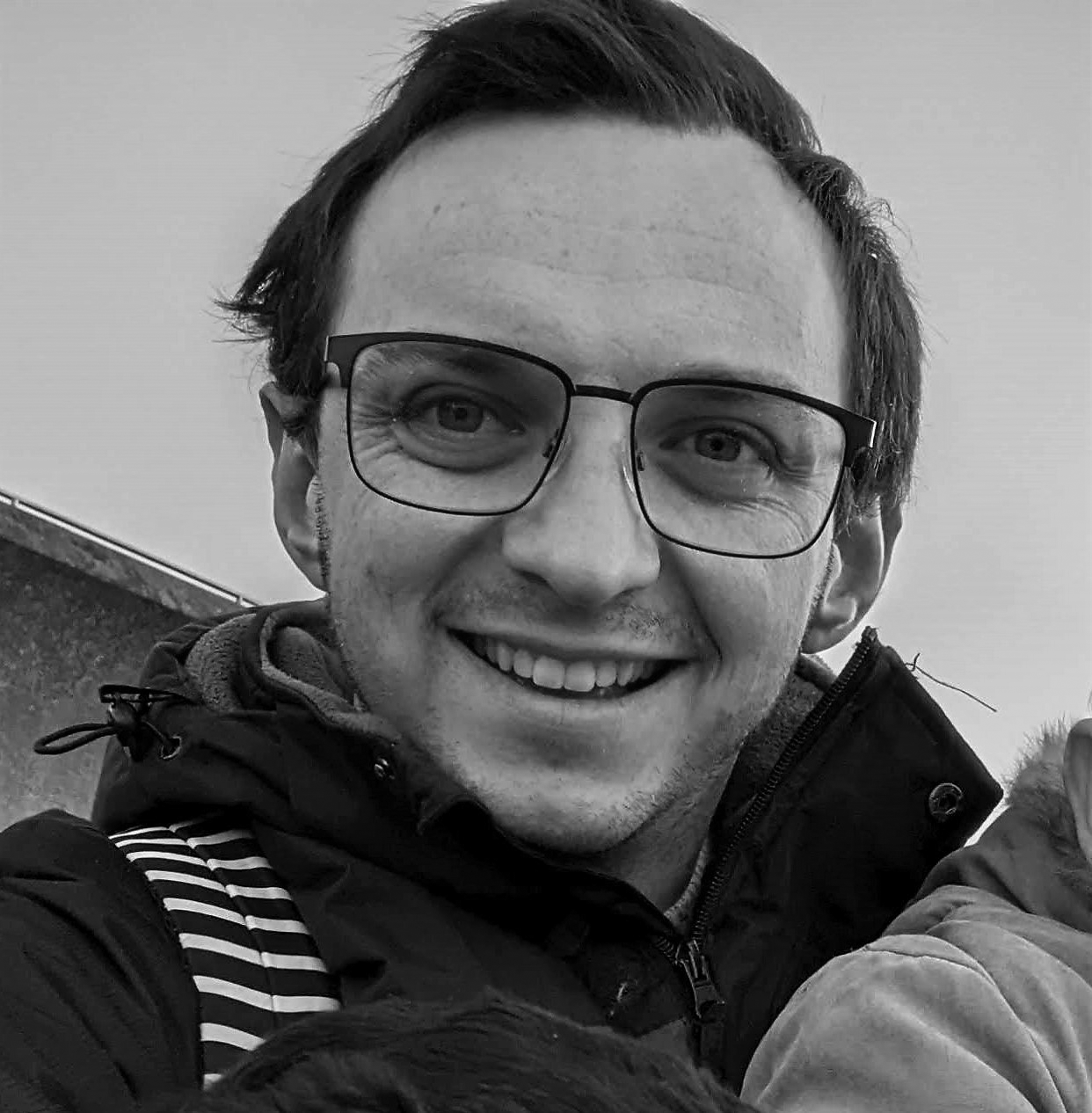
Author
Jamie WoodExpertise
Maths
Functions & Procedures in JavaScript
Functions and procedures are essential building blocks that allow you to enclose blocks of code and execute them as needed
They promote code reusability, modularity, and organisation, enabling a programmer to write efficient and maintainable programs
Considerations and Best Practices
Naming: Choose descriptive and meaningful names for your functions and procedures that indicate their purpose
Parameter Names: Use clear and meaningful parameter names to improve code readability
Function Length: Aim for functions and procedures that are short and focused
Return Values: Functions should have explicit return statements with meaningful return values, while procedures should not have return statements
Functions in JavaScript
A function is a reusable block of code that performs a specific task or calculation and can be called from anywhere in the code. Functions can take input parameters (arguments) and return a value.
The syntax for defining a function is as follows:
function functionName(parameter1, parameter2) {
// Code block to perform the task
// Return value;
}
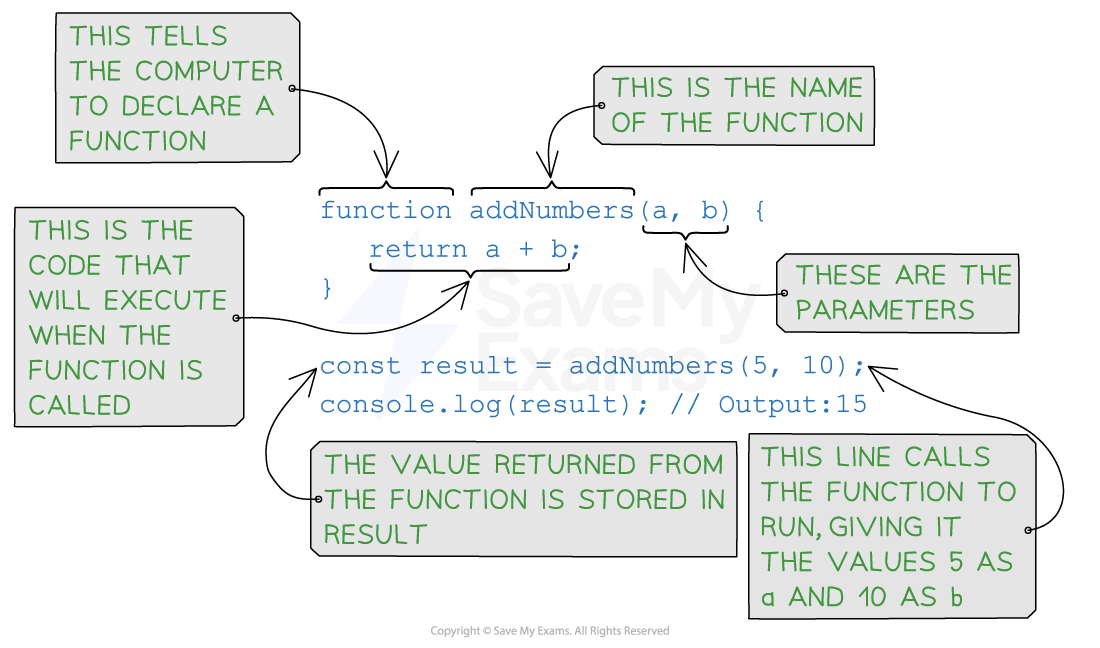
Function in JavaScript
Worked Example
A website sells tickets for sporting events. The website uses HTML, CSS and JavaScript. The website charges a booking fee of £2.99 on each ticket sold. In addition, if the tickets are purchased from outside of the UK, £4.99 is added to the booking fee. The booking fee is calculated using a JavaScript function named bookingfee()
.
Complete the definition of the bookingfee()
function below.function bookingfee(numtickets, country) {
var nonUKprice = 4.99;
var perTicketPrice = .............................................;
var total = 0;
if (country!="UK") {
total = total + .............................................;
}
total = total + (............................................. * perTicketPrice);
............................................. total;
}
4 marks
How to answer this question:
The first blank space is to set the value of the
perTicketPrice
. The question tells us this is £2.99The 2nd blank space is to add something to
total
if the country is not equal to UK. The question tells us that if the tickets are purchased from outside the UK, £4.99 is added to the booking fee. This is stored in thenonUKprice
variableThe 3rd blank space is something multiplied by
perTicketPrice
. The question tells us each ticket is £2.99 so we need to multiply theperTicketPrice
by the number of tickets (callednumtickets
)The 4th blank space is the last line of the function. As it's a function, a value must be returned. A value hasn't yet been returned in this function so
total
must be the value returned
Answer:
Example answer that gets full marks:
function bookingfee(numtickets, country) {
var nonUKprice = 4.99;
var perTicketPrice = 2.99;
var total = 0;
if (country!="UK") {
total = total + nonUKprice;
}
total = total + (numtickets * perTicketPrice);
return total;
}
Procedures in JavaScript
A procedure is similar to a function but does not return a value. Instead, it performs a series of actions or operations which could be anything the programmer wants the procedure to execute
A procedure is essentially a function without a
return
statement or with areturn
statement that has no value to return
The syntax is the same as for functions:
function procedureName(parameter1, parameter2) {
// Code block to perform actions
// No return statement or return with no value;
}
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?