Strings in JavaScript (OCR A Level Computer Science)
Revision Note
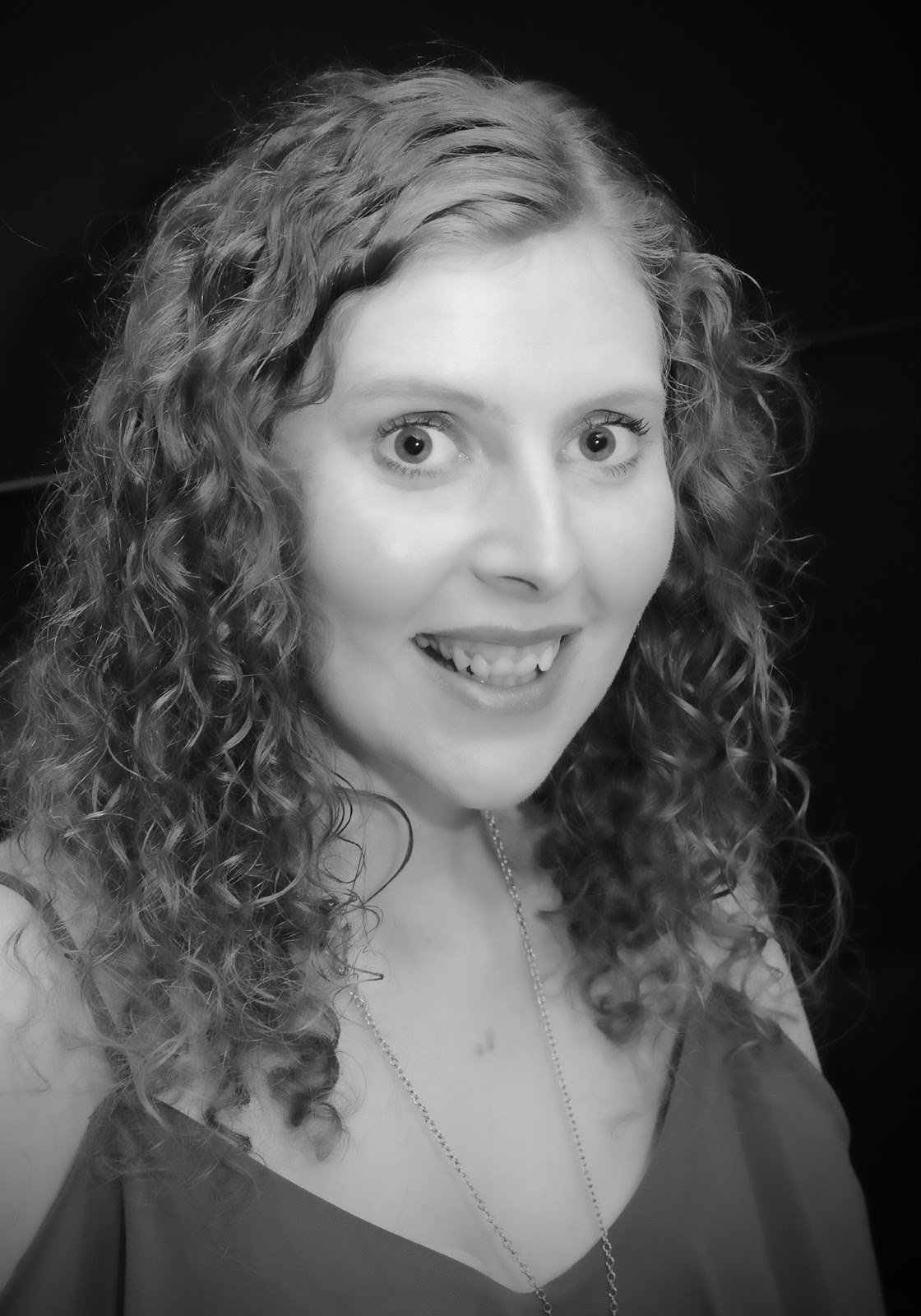
Author
Becci PetersExpertise
Computer Science
Strings in JavaScript
A string is a sequence of characters enclosed in single quotes ('
) or double quotes ("
). Strings are widely used for representing and manipulating text-based data.
Creating strings
Strings can be created in JavaScript by enclosing text within quotes:
const message = 'Hello, world!';
const name = "John Doe";
String length
To find the length of a string, use the
length
propertyThe
length
property returns the number of characters in a string:
const message = 'Hello, world!';
console.log(message.length);
// Output: 13
In this example, the
length
property is used to determine the number of characters in themessage
string
Substring extraction
To extract a portion of a string, use the
substring()
methodThe
substring()
method takes two parameters: the starting index and the ending index (optional)A new string is returned containing the characters within the specified range:
const message = 'Hello, world!';
const substring = message.substring(0, 5);
console.log(substring);
// Output: Hello
In this example, the
substring()
method is used to extract the characters from index 0 to index 5 (exclusive) from themessage
string, resulting in the substring'Hello'
By omitting the second parameter, the
substring()
method will extract the characters from the starting index to the end of the string:
const message = 'Hello, world!';
const substring = message.substring(7);
console.log(substring);
// Output: world!
Here, the
substring()
method extracts the characters from index 7 to the end of themessage
string, resulting in the substring'world!'
Exam Tip
JavaScript allows flexibility in choosing single or double quotes to define strings, as long as you maintain consistency within a string
There are many other things you can do with strings in JavaScript but these are the only ones you need to know before the exam
The exam might give you additional string functionality but if it does it will provide the code and explain it
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?