Linked Lists (OCR A Level Computer Science)
Revision Note
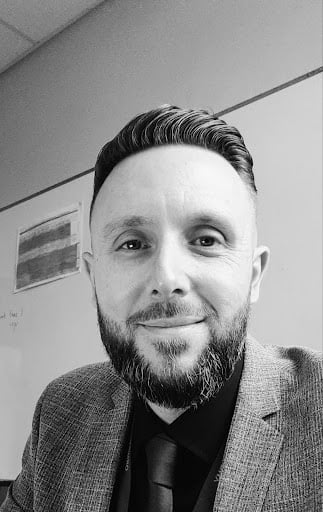
Author
Robert HamptonExpertise
Computer Science Content Creator
Implementing a Linked List
How do you Program a Linked List?
To recap the main operations of Linked Lists and how they work, follow this link
To program a Linked List you must be able to perform the following operations:
Create a Linked List
Traverse a Linked List
Add data to a Linked List
Remove data from a Linked List
Create a Linked List
Define the class called ‘Node’ that represents a node in the linked list.
Each node has 2 fields ‘fruit’ (this stores the fruit value) and ‘next’ (this stores a reference to the next node in the list)
We create 3 nodes and assign fruit values to each node
To establish a connection between the nodes we set the ‘next’ field of each node to the appropriate next node
Pseudocode |
|
Python |
|
Java |
|
Algorithm to Traverse a Linked List
We traverse the linked list starting from ‘node1’. We output the fruit value of each node and update the ‘current’ reference to the next node until we reach the end of the list
Pseudocode |
|
Python |
|
Java |
|
Algorithm to Add Data to a Linked List
We create a new node with the new data and check if the list is empty
If it isn’t, we find the last node and add the new node to the end
Pseudocode |
|
Python |
|
Java |
|
Algorithm to Remove Data from a Linked List
We traverse the list until we find the data to be removed
If the data to be removed is the first node, we update the head, else we update the node before to bypass the data to be removed
Pseudocode |
|
Python |
|
Java |
|
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?