Programming Encapsulation (OCR A Level Computer Science)
Revision Note
Programming Encapsulation
To program encapsulation, you should already have a solid understanding of what encapsulation in Object Oriented Programming (OOP) is.
How do you Define Encapsulation?
Get Methods
The "get" method is a common naming convention for a type of method that is used to retrieve the value of an object's private attributes (instance variables).
These methods are also known as "getter" methods or "accessor" methods.
The main purpose of a get method is to provide controlled access to the internal state (data) of an object without allowing direct modification.
Attributes are often declared as private to achieve encapsulation, so cannot be accessed directly from outside the class.
External code can use the public get methods to read the values of these private attributes (also known as instance variables).
Pseudocode
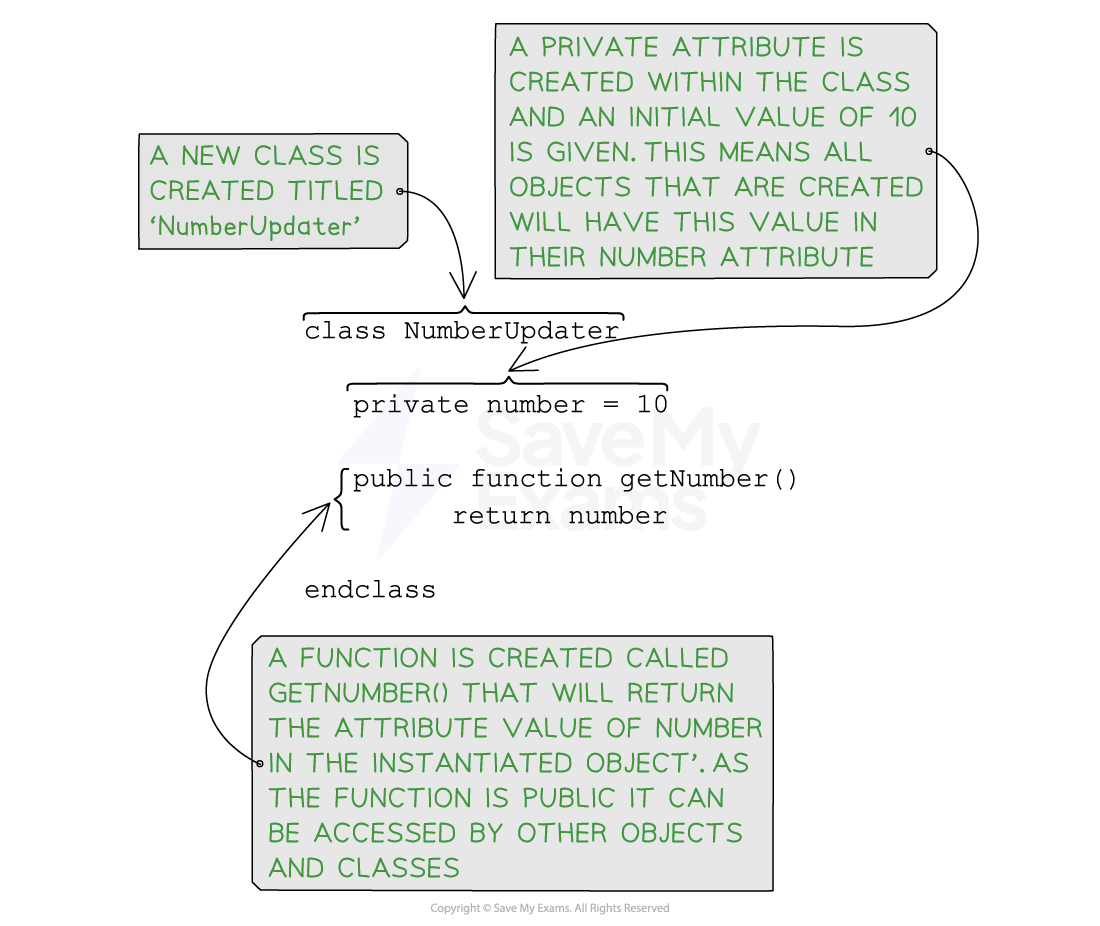
Pseudocode for a get method
Java
//creating a class called NumberUpdater
public class NumberUpdater {
//setting the private attribute to the value of 10
private int number = 10;
//creating a get method that will return the value store in the number attribute
public int getNumber() {
return number;
}
}
Python
#creating a class called NumberUpdater
class NumberUpdater:
#setting the private attribute to the value of 10
def __init__(self):
self.__number = 10
#creating a get method that will return the value stored in the number attribute
@property
def number(self):
return self.__number
Set Methods
The "set" method is a common naming convention for a type of method that is used to set the value of object's private instance variables.
Setter methods, also known as “setter” methods or “mutator” methods
Generally additional code is written within the method to allow controlled access by enforcing certain conditions or validations before updating the attribute
Pseudocode
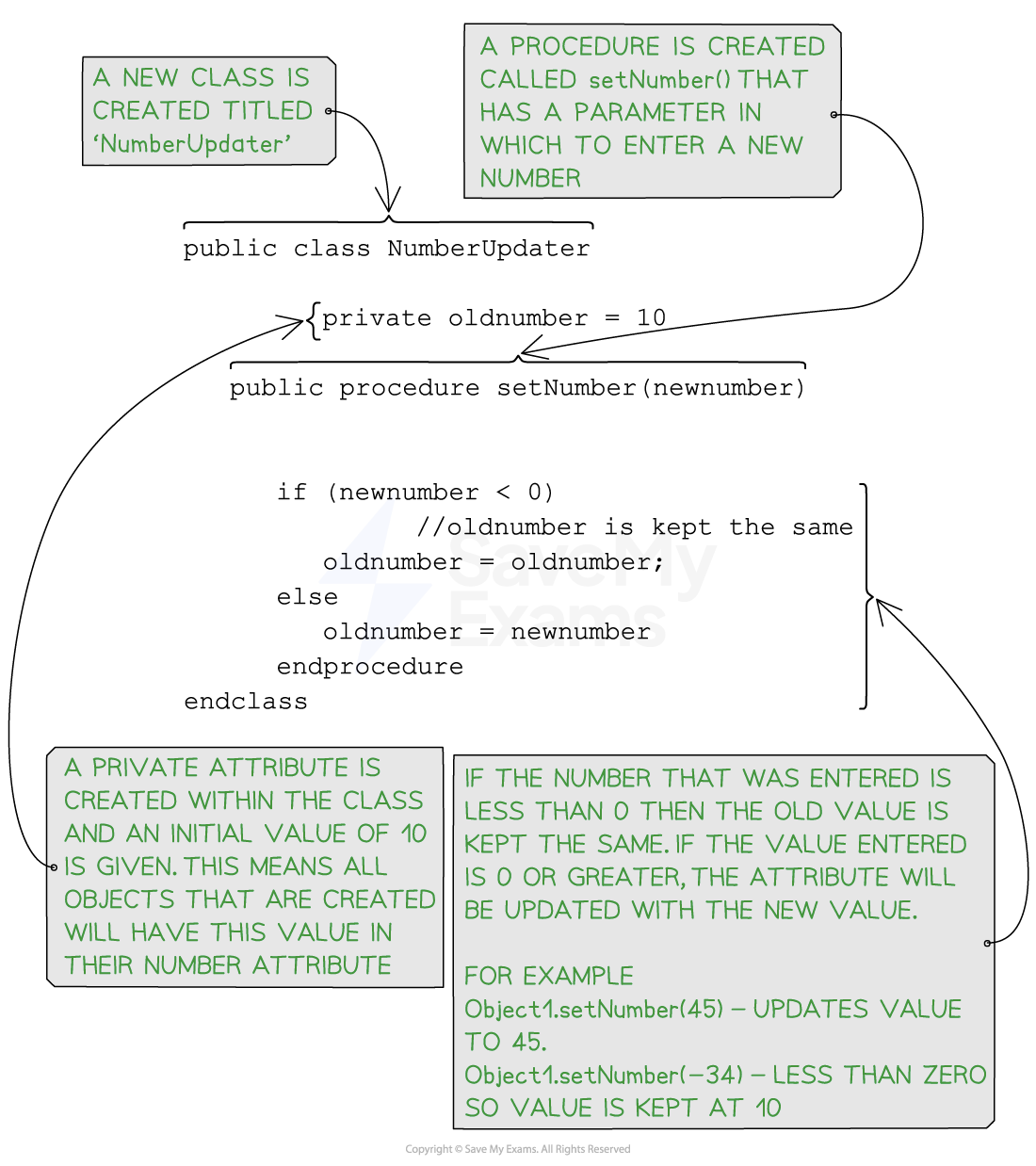
Pseudocode for a "setter" method
Java
//creating a class called NumberUpdater
public class NumberUpdater
//setting attribute value to 10
private int oldnumber = 10;
//creating a set method
public void setNumber(int newnumber) {
//if the new number is less than zero
if (newnumber < 0) {
//oldnumber is kept the same
oldnumber = oldnumber;
} else {
//oldnumber is updated with the new number value
oldnumber = newnumber;
}
}
}
Python
//creating a class called NumberUpdater
class NumberUpdater:
//setting attribute value to 10
def __init__(self):
self.__oldnumber = 10
//creating a set method
def setNumber(self, newnumber):
//if the new number is less than zero
if newnumber < 0:
//oldnumber is kept the same
self.__oldnumber = self.__oldnumber
else:
//oldnumber is updated with the new number value
self.__oldnumber = newnumber
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?